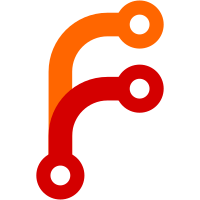
2005-02-21 Andrew Haley <aph@redhat.com> * Makefile.in: Rebuilt. * Makefile.am (nat_source_files): Added natLogger.cc. * java/util/logging/natLogger.cc: New file. * java/util/logging/Logger.java (getCallerStackFrame): Now native. From-SVN: r95338
55 lines
1.3 KiB
C++
55 lines
1.3 KiB
C++
// natLogger.cc - Native part of Logger class.
|
|
|
|
/* Copyright (C) 1998, 1999, 2000, 2001 Free Software Foundation
|
|
|
|
This Logger is part of libgcj.
|
|
|
|
This software is copyrighted work licensed under the terms of the
|
|
Libgcj License. Please consult the Logger "LIBGCJ_LICENSE" for
|
|
details. */
|
|
|
|
#include <config.h>
|
|
#include <platform.h>
|
|
|
|
#include <string.h>
|
|
|
|
#pragma implementation "Logger.h"
|
|
|
|
#include <gcj/cni.h>
|
|
#include <jvm.h>
|
|
|
|
|
|
#include <java/lang/Object.h>
|
|
#include <java/lang/Class.h>
|
|
#include <java/util/logging/Logger.h>
|
|
#include <java/lang/StackTraceElement.h>
|
|
#include <java/lang/ArrayIndexOutOfBoundsException.h>
|
|
|
|
java::lang::StackTraceElement*
|
|
java::util::logging::Logger::getCallerStackFrame ()
|
|
{
|
|
gnu::gcj::runtime::StackTrace *t
|
|
= new gnu::gcj::runtime::StackTrace(4);
|
|
java::lang::Class *klass = NULL;
|
|
int i = 2;
|
|
try
|
|
{
|
|
// skip until this class
|
|
while ((klass = t->classAt (i)) != getClass())
|
|
i++;
|
|
// skip the stackentries of this class
|
|
while ((klass = t->classAt (i)) == getClass() || klass == NULL)
|
|
i++;
|
|
}
|
|
catch (::java::lang::ArrayIndexOutOfBoundsException *e)
|
|
{
|
|
// FIXME: RuntimeError
|
|
}
|
|
|
|
java::lang::StackTraceElement *e
|
|
= new java::lang::StackTraceElement
|
|
(JvNewStringUTF (""), 0,
|
|
klass->getName(), t->methodAt(i), false);
|
|
|
|
return e;
|
|
}
|