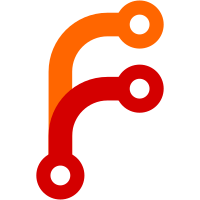
P1008R1 - prohibit aggregates with user-declared constructors * class.c (check_bases_and_members): For C++2a set CLASSTYPE_NON_AGGREGATE based on TYPE_HAS_USER_CONSTRUCTOR rather than type_has_user_provided_or_explicit_constructor. * g++.dg/ext/is_aggregate.C: Add tests with deleted or defaulted ctor. * g++.dg/cpp0x/defaulted1.C (main): Ifdef out for C++2a B b = {1};. * g++.dg/cpp0x/deleted2.C: Expect error for C++2a. * g++.dg/cpp2a/aggr1.C: New test. * g++.dg/cpp2a/aggr2.C: New test. From-SVN: r263115
44 lines
528 B
C
44 lines
528 B
C
// Positive test for defaulted/deleted fns
|
|
// { dg-do run { target c++11 } }
|
|
|
|
struct A
|
|
{
|
|
int i;
|
|
A() = default;
|
|
A(const A&) = delete;
|
|
A& operator=(const A&) = default;
|
|
~A();
|
|
};
|
|
|
|
A::~A() = default;
|
|
|
|
void f() = delete;
|
|
|
|
struct B
|
|
{
|
|
int i;
|
|
B() = default;
|
|
};
|
|
|
|
int main()
|
|
{
|
|
A a1, a2;
|
|
#if __cplusplus <= 201703L
|
|
B b = {1};
|
|
#endif
|
|
a1 = a2;
|
|
}
|
|
|
|
// fns defaulted in class defn are trivial
|
|
struct C
|
|
{
|
|
C() = default;
|
|
C(const C&) = default;
|
|
C& operator=(const C&) = default;
|
|
~C() = default;
|
|
};
|
|
|
|
union U
|
|
{
|
|
C c;
|
|
};
|