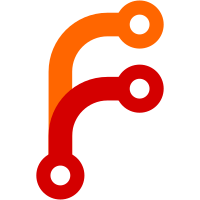
This implements the C++23 paper P2419R2 (Clarify handling of encodings in localized formatting of chrono types). The requirement is that when the literal encoding is "a Unicode encoding form" and the formatting locale uses a different encoding, any locale-specific strings such as "août" for std::chrono::August should be converted to the literal encoding. Using the recently-added std::locale::encoding() function we can check the locale's encoding and then use iconv if a conversion is needed. Because nl_langinfo_l and iconv_open both allocate memory, a naive implementation would perform multiple allocations and deallocations for every snippet of locale-specific text that needs to be converted to UTF-8. To avoid that, a new internal locale::facet is defined to store the text_encoding and an iconv_t descriptor, which are then cached in the formatting locale. This requires access to the internals of a std::locale object in src/c++20/format.cc, so that new file needs to be compiled with -fno-access-control, as well as -std=gnu++26 in order to use std::text_encoding. Because the new std::text_encoding and std::locale::encoding() symbols are only in the libstdc++exp.a archive, we need to include src/c++26/text_encoding.cc in the main library, but not export its symbols yet. This means they can be used by the two new functions which are exported from the main library. The encoding conversions are done for C++20, treating it as a DR that resolves LWG 3656. With this change we can increase the value of the __cpp_lib_format macro for C++23. The value should be 202207 for P2419R2, but we already implement P2510R3 (Formatting pointers) so can use the value 202304. libstdc++-v3/ChangeLog: PR libstdc++/109162 * acinclude.m4 (libtool_VERSION): Update to 6:34:0. * config/abi/pre/gnu.ver: Disambiguate old patters. Add new GLIBCXX_3.4.34 symbol version and new exports. * configure: Regenerate. * include/bits/chrono_io.h (_ChronoSpec::_M_locale_specific): Add new accessor functions to use a reserved bit in _Spec. (__formatter_chrono::_M_parse): Use _M_locale_specific(true) when chrono-specs contains locale-dependent conversion specifiers. (__formatter_chrono::_M_format): Open iconv descriptor if conversion to UTF-8 will be needed. (__formatter_chrono::_M_write): New function to write a localized string with possible character conversion. (__formatter_chrono::_M_a_A, __formatter_chrono::_M_b_B) (__formatter_chrono::_M_p, __formatter_chrono::_M_r) (__formatter_chrono::_M_x, __formatter_chrono::_M_X) (__formatter_chrono::_M_locale_fmt): Use _M_write. * include/bits/version.def (format): Update value. * include/bits/version.h: Regenerate. * include/std/format (_GLIBCXX_P2518R3): Check feature test macro instead of __cplusplus. (basic_format_context): Declare __formatter_chrono as friend. * src/c++20/Makefile.am: Add new file. * src/c++20/Makefile.in: Regenerate. * src/c++20/format.cc: New file. * testsuite/std/time/format_localized.cc: New test. * testsuite/util/testsuite_abi.cc: Add new symbol version.
2026 lines
80 KiB
C++
2026 lines
80 KiB
C++
// Copyright (C) 2023-2024 Free Software Foundation, Inc.
|
|
|
|
// This file is part of the GNU ISO C++ Library. This library is free
|
|
// software; you can redistribute it and/or modify it under the
|
|
// terms of the GNU General Public License as published by the
|
|
// Free Software Foundation; either version 3, or (at your option)
|
|
// any later version.
|
|
|
|
// This library is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
// GNU General Public License for more details.
|
|
|
|
// Under Section 7 of GPL version 3, you are granted additional
|
|
// permissions described in the GCC Runtime Library Exception, version
|
|
// 3.1, as published by the Free Software Foundation.
|
|
|
|
// You should have received a copy of the GNU General Public License and
|
|
// a copy of the GCC Runtime Library Exception along with this program;
|
|
// see the files COPYING3 and COPYING.RUNTIME respectively. If not, see
|
|
// <http://www.gnu.org/licenses/>.
|
|
|
|
// DO NOT EDIT THIS FILE (version.h)
|
|
//
|
|
// It has been AutoGen-ed
|
|
// From the definitions version.def
|
|
// and the template file version.tpl
|
|
|
|
/** @file bits/version.h
|
|
* This is an internal header file, included by other library headers.
|
|
* Do not attempt to use it directly. @headername{version}
|
|
*/
|
|
|
|
// Usage guide:
|
|
//
|
|
// In your usual header, do something like:
|
|
//
|
|
// #define __glibcxx_want_ranges
|
|
// #define __glibcxx_want_concepts
|
|
// #include <bits/version.h>
|
|
//
|
|
// This will generate the FTMs you named, and let you use them in your code as
|
|
// if it was user code. All macros are also exposed under __glibcxx_NAME even
|
|
// if unwanted, to permit bits and other FTMs to depend on them for condtional
|
|
// computation without exposing extra FTMs to user code.
|
|
|
|
#pragma GCC system_header
|
|
|
|
#include <bits/c++config.h>
|
|
|
|
#if !defined(__cpp_lib_incomplete_container_elements)
|
|
# if _GLIBCXX_HOSTED
|
|
# define __glibcxx_incomplete_container_elements 201505L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_incomplete_container_elements)
|
|
# define __cpp_lib_incomplete_container_elements 201505L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_incomplete_container_elements) && defined(__glibcxx_want_incomplete_container_elements) */
|
|
#undef __glibcxx_want_incomplete_container_elements
|
|
|
|
#if !defined(__cpp_lib_uncaught_exceptions)
|
|
# if ((defined(__STRICT_ANSI__) && __cplusplus >= 201703L) || (!defined(__STRICT_ANSI__) && __cplusplus >= 199711L))
|
|
# define __glibcxx_uncaught_exceptions 201411L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_uncaught_exceptions)
|
|
# define __cpp_lib_uncaught_exceptions 201411L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_uncaught_exceptions) && defined(__glibcxx_want_uncaught_exceptions) */
|
|
#undef __glibcxx_want_uncaught_exceptions
|
|
|
|
#if !defined(__cpp_lib_allocator_traits_is_always_equal)
|
|
# if (__cplusplus >= 201103L)
|
|
# define __glibcxx_allocator_traits_is_always_equal 201411L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_allocator_traits_is_always_equal)
|
|
# define __cpp_lib_allocator_traits_is_always_equal 201411L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_allocator_traits_is_always_equal) && defined(__glibcxx_want_allocator_traits_is_always_equal) */
|
|
#undef __glibcxx_want_allocator_traits_is_always_equal
|
|
|
|
#if !defined(__cpp_lib_is_null_pointer)
|
|
# if (__cplusplus >= 201103L)
|
|
# define __glibcxx_is_null_pointer 201309L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_null_pointer)
|
|
# define __cpp_lib_is_null_pointer 201309L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_null_pointer) && defined(__glibcxx_want_is_null_pointer) */
|
|
#undef __glibcxx_want_is_null_pointer
|
|
|
|
#if !defined(__cpp_lib_result_of_sfinae)
|
|
# if (__cplusplus >= 201103L)
|
|
# define __glibcxx_result_of_sfinae 201210L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_result_of_sfinae)
|
|
# define __cpp_lib_result_of_sfinae 201210L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_result_of_sfinae) && defined(__glibcxx_want_result_of_sfinae) */
|
|
#undef __glibcxx_want_result_of_sfinae
|
|
|
|
#if !defined(__cpp_lib_shared_ptr_arrays)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_shared_ptr_arrays 201707L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_shared_ptr_arrays)
|
|
# define __cpp_lib_shared_ptr_arrays 201707L
|
|
# endif
|
|
# elif (__cplusplus >= 201103L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_shared_ptr_arrays 201611L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_shared_ptr_arrays)
|
|
# define __cpp_lib_shared_ptr_arrays 201611L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_shared_ptr_arrays) && defined(__glibcxx_want_shared_ptr_arrays) */
|
|
#undef __glibcxx_want_shared_ptr_arrays
|
|
|
|
#if !defined(__cpp_lib_is_swappable)
|
|
# if ((defined(__STRICT_ANSI__) && __cplusplus >= 201703L) || (!defined(__STRICT_ANSI__) && __cplusplus >= 201103L))
|
|
# define __glibcxx_is_swappable 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_swappable)
|
|
# define __cpp_lib_is_swappable 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_swappable) && defined(__glibcxx_want_is_swappable) */
|
|
#undef __glibcxx_want_is_swappable
|
|
|
|
#if !defined(__cpp_lib_void_t)
|
|
# if ((defined(__STRICT_ANSI__) && __cplusplus >= 201703L) || (!defined(__STRICT_ANSI__) && __cplusplus >= 201103L))
|
|
# define __glibcxx_void_t 201411L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_void_t)
|
|
# define __cpp_lib_void_t 201411L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_void_t) && defined(__glibcxx_want_void_t) */
|
|
#undef __glibcxx_want_void_t
|
|
|
|
#if !defined(__cpp_lib_enable_shared_from_this)
|
|
# if ((defined(__STRICT_ANSI__) && __cplusplus >= 201703L) || (!defined(__STRICT_ANSI__) && __cplusplus >= 201103L)) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_enable_shared_from_this 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_enable_shared_from_this)
|
|
# define __cpp_lib_enable_shared_from_this 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_enable_shared_from_this) && defined(__glibcxx_want_enable_shared_from_this) */
|
|
#undef __glibcxx_want_enable_shared_from_this
|
|
|
|
#if !defined(__cpp_lib_math_spec_funcs)
|
|
# if (__cplusplus >= 201103L)
|
|
# define __glibcxx_math_spec_funcs 201003L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_math_spec_funcs)
|
|
# define __STDCPP_MATH_SPEC_FUNCS__ 201003L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_math_spec_funcs) && defined(__glibcxx_want_math_spec_funcs) */
|
|
#undef __glibcxx_want_math_spec_funcs
|
|
|
|
#if !defined(__cpp_lib_coroutine)
|
|
# if (__cplusplus >= 201402L) && (__cpp_impl_coroutine)
|
|
# define __glibcxx_coroutine 201902L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_coroutine)
|
|
# define __cpp_lib_coroutine 201902L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_coroutine) && defined(__glibcxx_want_coroutine) */
|
|
#undef __glibcxx_want_coroutine
|
|
|
|
#if !defined(__cpp_lib_exchange_function)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_exchange_function 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_exchange_function)
|
|
# define __cpp_lib_exchange_function 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_exchange_function) && defined(__glibcxx_want_exchange_function) */
|
|
#undef __glibcxx_want_exchange_function
|
|
|
|
#if !defined(__cpp_lib_integer_sequence)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_integer_sequence 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_integer_sequence)
|
|
# define __cpp_lib_integer_sequence 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_integer_sequence) && defined(__glibcxx_want_integer_sequence) */
|
|
#undef __glibcxx_want_integer_sequence
|
|
|
|
#if !defined(__cpp_lib_integral_constant_callable)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_integral_constant_callable 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_integral_constant_callable)
|
|
# define __cpp_lib_integral_constant_callable 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_integral_constant_callable) && defined(__glibcxx_want_integral_constant_callable) */
|
|
#undef __glibcxx_want_integral_constant_callable
|
|
|
|
#if !defined(__cpp_lib_is_final)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_is_final 201402L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_final)
|
|
# define __cpp_lib_is_final 201402L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_final) && defined(__glibcxx_want_is_final) */
|
|
#undef __glibcxx_want_is_final
|
|
|
|
#if !defined(__cpp_lib_make_reverse_iterator)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_make_reverse_iterator 201402L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_make_reverse_iterator)
|
|
# define __cpp_lib_make_reverse_iterator 201402L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_make_reverse_iterator) && defined(__glibcxx_want_make_reverse_iterator) */
|
|
#undef __glibcxx_want_make_reverse_iterator
|
|
|
|
#if !defined(__cpp_lib_null_iterators)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_null_iterators 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_null_iterators)
|
|
# define __cpp_lib_null_iterators 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_null_iterators) && defined(__glibcxx_want_null_iterators) */
|
|
#undef __glibcxx_want_null_iterators
|
|
|
|
#if !defined(__cpp_lib_transformation_trait_aliases)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_transformation_trait_aliases 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_transformation_trait_aliases)
|
|
# define __cpp_lib_transformation_trait_aliases 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_transformation_trait_aliases) && defined(__glibcxx_want_transformation_trait_aliases) */
|
|
#undef __glibcxx_want_transformation_trait_aliases
|
|
|
|
#if !defined(__cpp_lib_transparent_operators)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_transparent_operators 201510L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_transparent_operators)
|
|
# define __cpp_lib_transparent_operators 201510L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_transparent_operators) && defined(__glibcxx_want_transparent_operators) */
|
|
#undef __glibcxx_want_transparent_operators
|
|
|
|
#if !defined(__cpp_lib_tuple_element_t)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_tuple_element_t 201402L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_tuple_element_t)
|
|
# define __cpp_lib_tuple_element_t 201402L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_tuple_element_t) && defined(__glibcxx_want_tuple_element_t) */
|
|
#undef __glibcxx_want_tuple_element_t
|
|
|
|
#if !defined(__cpp_lib_tuples_by_type)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_tuples_by_type 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_tuples_by_type)
|
|
# define __cpp_lib_tuples_by_type 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_tuples_by_type) && defined(__glibcxx_want_tuples_by_type) */
|
|
#undef __glibcxx_want_tuples_by_type
|
|
|
|
#if !defined(__cpp_lib_robust_nonmodifying_seq_ops)
|
|
# if (__cplusplus >= 201402L)
|
|
# define __glibcxx_robust_nonmodifying_seq_ops 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_robust_nonmodifying_seq_ops)
|
|
# define __cpp_lib_robust_nonmodifying_seq_ops 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_robust_nonmodifying_seq_ops) && defined(__glibcxx_want_robust_nonmodifying_seq_ops) */
|
|
#undef __glibcxx_want_robust_nonmodifying_seq_ops
|
|
|
|
#if !defined(__cpp_lib_to_chars)
|
|
# if (__cplusplus > 202302L) && (_GLIBCXX_FLOAT_IS_IEEE_BINARY32 && _GLIBCXX_DOUBLE_IS_IEEE_BINARY64 && __SIZE_WIDTH__ >= 32)
|
|
# define __glibcxx_to_chars 202306L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_to_chars)
|
|
# define __cpp_lib_to_chars 202306L
|
|
# endif
|
|
# elif (__cplusplus >= 201402L) && (_GLIBCXX_FLOAT_IS_IEEE_BINARY32 && _GLIBCXX_DOUBLE_IS_IEEE_BINARY64 && __SIZE_WIDTH__ >= 32)
|
|
# define __glibcxx_to_chars 201611L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_to_chars)
|
|
# define __cpp_lib_to_chars 201611L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_to_chars) && defined(__glibcxx_want_to_chars) */
|
|
#undef __glibcxx_want_to_chars
|
|
|
|
#if !defined(__cpp_lib_chrono_udls)
|
|
# if (__cplusplus >= 201402L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_chrono_udls 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_chrono_udls)
|
|
# define __cpp_lib_chrono_udls 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_chrono_udls) && defined(__glibcxx_want_chrono_udls) */
|
|
#undef __glibcxx_want_chrono_udls
|
|
|
|
#if !defined(__cpp_lib_complex_udls)
|
|
# if (__cplusplus >= 201402L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_complex_udls 201309L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_complex_udls)
|
|
# define __cpp_lib_complex_udls 201309L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_complex_udls) && defined(__glibcxx_want_complex_udls) */
|
|
#undef __glibcxx_want_complex_udls
|
|
|
|
#if !defined(__cpp_lib_generic_associative_lookup)
|
|
# if (__cplusplus >= 201402L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_generic_associative_lookup 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_generic_associative_lookup)
|
|
# define __cpp_lib_generic_associative_lookup 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_generic_associative_lookup) && defined(__glibcxx_want_generic_associative_lookup) */
|
|
#undef __glibcxx_want_generic_associative_lookup
|
|
|
|
#if !defined(__cpp_lib_make_unique)
|
|
# if (__cplusplus >= 201402L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_make_unique 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_make_unique)
|
|
# define __cpp_lib_make_unique 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_make_unique) && defined(__glibcxx_want_make_unique) */
|
|
#undef __glibcxx_want_make_unique
|
|
|
|
#if !defined(__cpp_lib_quoted_string_io)
|
|
# if (__cplusplus >= 201402L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_quoted_string_io 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_quoted_string_io)
|
|
# define __cpp_lib_quoted_string_io 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_quoted_string_io) && defined(__glibcxx_want_quoted_string_io) */
|
|
#undef __glibcxx_want_quoted_string_io
|
|
|
|
#if !defined(__cpp_lib_shared_timed_mutex)
|
|
# if (__cplusplus >= 201402L) && defined(_GLIBCXX_HAS_GTHREADS) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_shared_timed_mutex 201402L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_shared_timed_mutex)
|
|
# define __cpp_lib_shared_timed_mutex 201402L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_shared_timed_mutex) && defined(__glibcxx_want_shared_timed_mutex) */
|
|
#undef __glibcxx_want_shared_timed_mutex
|
|
|
|
#if !defined(__cpp_lib_string_udls)
|
|
# if (__cplusplus >= 201402L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_string_udls 201304L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_string_udls)
|
|
# define __cpp_lib_string_udls 201304L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_string_udls) && defined(__glibcxx_want_string_udls) */
|
|
#undef __glibcxx_want_string_udls
|
|
|
|
#if !defined(__cpp_lib_addressof_constexpr)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_addressof_constexpr 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_addressof_constexpr)
|
|
# define __cpp_lib_addressof_constexpr 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_addressof_constexpr) && defined(__glibcxx_want_addressof_constexpr) */
|
|
#undef __glibcxx_want_addressof_constexpr
|
|
|
|
#if !defined(__cpp_lib_any)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_any 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_any)
|
|
# define __cpp_lib_any 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_any) && defined(__glibcxx_want_any) */
|
|
#undef __glibcxx_want_any
|
|
|
|
#if !defined(__cpp_lib_apply)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_apply 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_apply)
|
|
# define __cpp_lib_apply 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_apply) && defined(__glibcxx_want_apply) */
|
|
#undef __glibcxx_want_apply
|
|
|
|
#if !defined(__cpp_lib_as_const)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_as_const 201510L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_as_const)
|
|
# define __cpp_lib_as_const 201510L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_as_const) && defined(__glibcxx_want_as_const) */
|
|
#undef __glibcxx_want_as_const
|
|
|
|
#if !defined(__cpp_lib_atomic_is_always_lock_free)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_atomic_is_always_lock_free 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_atomic_is_always_lock_free)
|
|
# define __cpp_lib_atomic_is_always_lock_free 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_atomic_is_always_lock_free) && defined(__glibcxx_want_atomic_is_always_lock_free) */
|
|
#undef __glibcxx_want_atomic_is_always_lock_free
|
|
|
|
#if !defined(__cpp_lib_bool_constant)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_bool_constant 201505L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_bool_constant)
|
|
# define __cpp_lib_bool_constant 201505L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_bool_constant) && defined(__glibcxx_want_bool_constant) */
|
|
#undef __glibcxx_want_bool_constant
|
|
|
|
#if !defined(__cpp_lib_byte)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_byte 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_byte)
|
|
# define __cpp_lib_byte 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_byte) && defined(__glibcxx_want_byte) */
|
|
#undef __glibcxx_want_byte
|
|
|
|
#if !defined(__cpp_lib_has_unique_object_representations)
|
|
# if (__cplusplus >= 201703L) && (defined(_GLIBCXX_HAVE_BUILTIN_HAS_UNIQ_OBJ_REP))
|
|
# define __glibcxx_has_unique_object_representations 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_has_unique_object_representations)
|
|
# define __cpp_lib_has_unique_object_representations 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_has_unique_object_representations) && defined(__glibcxx_want_has_unique_object_representations) */
|
|
#undef __glibcxx_want_has_unique_object_representations
|
|
|
|
#if !defined(__cpp_lib_hardware_interference_size)
|
|
# if (__cplusplus >= 201703L) && (defined(__GCC_DESTRUCTIVE_SIZE))
|
|
# define __glibcxx_hardware_interference_size 201703L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_hardware_interference_size)
|
|
# define __cpp_lib_hardware_interference_size 201703L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_hardware_interference_size) && defined(__glibcxx_want_hardware_interference_size) */
|
|
#undef __glibcxx_want_hardware_interference_size
|
|
|
|
#if !defined(__cpp_lib_invoke)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_invoke 201411L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_invoke)
|
|
# define __cpp_lib_invoke 201411L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_invoke) && defined(__glibcxx_want_invoke) */
|
|
#undef __glibcxx_want_invoke
|
|
|
|
#if !defined(__cpp_lib_is_aggregate)
|
|
# if (__cplusplus >= 201703L) && (defined(_GLIBCXX_HAVE_BUILTIN_IS_AGGREGATE))
|
|
# define __glibcxx_is_aggregate 201703L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_aggregate)
|
|
# define __cpp_lib_is_aggregate 201703L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_aggregate) && defined(__glibcxx_want_is_aggregate) */
|
|
#undef __glibcxx_want_is_aggregate
|
|
|
|
#if !defined(__cpp_lib_is_invocable)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_is_invocable 201703L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_invocable)
|
|
# define __cpp_lib_is_invocable 201703L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_invocable) && defined(__glibcxx_want_is_invocable) */
|
|
#undef __glibcxx_want_is_invocable
|
|
|
|
#if !defined(__cpp_lib_launder)
|
|
# if (__cplusplus >= 201703L) && (defined(_GLIBCXX_HAVE_BUILTIN_LAUNDER))
|
|
# define __glibcxx_launder 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_launder)
|
|
# define __cpp_lib_launder 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_launder) && defined(__glibcxx_want_launder) */
|
|
#undef __glibcxx_want_launder
|
|
|
|
#if !defined(__cpp_lib_logical_traits)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_logical_traits 201510L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_logical_traits)
|
|
# define __cpp_lib_logical_traits 201510L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_logical_traits) && defined(__glibcxx_want_logical_traits) */
|
|
#undef __glibcxx_want_logical_traits
|
|
|
|
#if !defined(__cpp_lib_make_from_tuple)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_make_from_tuple 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_make_from_tuple)
|
|
# define __cpp_lib_make_from_tuple 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_make_from_tuple) && defined(__glibcxx_want_make_from_tuple) */
|
|
#undef __glibcxx_want_make_from_tuple
|
|
|
|
#if !defined(__cpp_lib_not_fn)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_not_fn 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_not_fn)
|
|
# define __cpp_lib_not_fn 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_not_fn) && defined(__glibcxx_want_not_fn) */
|
|
#undef __glibcxx_want_not_fn
|
|
|
|
#if !defined(__cpp_lib_type_trait_variable_templates)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_type_trait_variable_templates 201510L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_type_trait_variable_templates)
|
|
# define __cpp_lib_type_trait_variable_templates 201510L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_type_trait_variable_templates) && defined(__glibcxx_want_type_trait_variable_templates) */
|
|
#undef __glibcxx_want_type_trait_variable_templates
|
|
|
|
#if !defined(__cpp_lib_variant)
|
|
# if (__cplusplus >= 202002L) && (__cpp_concepts >= 202002L && __cpp_constexpr >= 201811L)
|
|
# define __glibcxx_variant 202106L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_variant)
|
|
# define __cpp_lib_variant 202106L
|
|
# endif
|
|
# elif (__cplusplus >= 201703L)
|
|
# define __glibcxx_variant 202102L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_variant)
|
|
# define __cpp_lib_variant 202102L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_variant) && defined(__glibcxx_want_variant) */
|
|
#undef __glibcxx_want_variant
|
|
|
|
#if !defined(__cpp_lib_lcm)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_lcm 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_lcm)
|
|
# define __cpp_lib_lcm 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_lcm) && defined(__glibcxx_want_lcm) */
|
|
#undef __glibcxx_want_lcm
|
|
|
|
#if !defined(__cpp_lib_gcd)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_gcd 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_gcd)
|
|
# define __cpp_lib_gcd 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_gcd) && defined(__glibcxx_want_gcd) */
|
|
#undef __glibcxx_want_gcd
|
|
|
|
#if !defined(__cpp_lib_gcd_lcm)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_gcd_lcm 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_gcd_lcm)
|
|
# define __cpp_lib_gcd_lcm 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_gcd_lcm) && defined(__glibcxx_want_gcd_lcm) */
|
|
#undef __glibcxx_want_gcd_lcm
|
|
|
|
#if !defined(__cpp_lib_raw_memory_algorithms)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_raw_memory_algorithms 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_raw_memory_algorithms)
|
|
# define __cpp_lib_raw_memory_algorithms 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_raw_memory_algorithms) && defined(__glibcxx_want_raw_memory_algorithms) */
|
|
#undef __glibcxx_want_raw_memory_algorithms
|
|
|
|
#if !defined(__cpp_lib_array_constexpr)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_array_constexpr 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_array_constexpr)
|
|
# define __cpp_lib_array_constexpr 201811L
|
|
# endif
|
|
# elif (__cplusplus >= 201703L)
|
|
# define __glibcxx_array_constexpr 201803L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_array_constexpr)
|
|
# define __cpp_lib_array_constexpr 201803L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_array_constexpr) && defined(__glibcxx_want_array_constexpr) */
|
|
#undef __glibcxx_want_array_constexpr
|
|
|
|
#if !defined(__cpp_lib_nonmember_container_access)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_nonmember_container_access 201411L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_nonmember_container_access)
|
|
# define __cpp_lib_nonmember_container_access 201411L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_nonmember_container_access) && defined(__glibcxx_want_nonmember_container_access) */
|
|
#undef __glibcxx_want_nonmember_container_access
|
|
|
|
#if !defined(__cpp_lib_clamp)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_clamp 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_clamp)
|
|
# define __cpp_lib_clamp 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_clamp) && defined(__glibcxx_want_clamp) */
|
|
#undef __glibcxx_want_clamp
|
|
|
|
#if !defined(__cpp_lib_sample)
|
|
# if (__cplusplus >= 201703L)
|
|
# define __glibcxx_sample 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_sample)
|
|
# define __cpp_lib_sample 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_sample) && defined(__glibcxx_want_sample) */
|
|
#undef __glibcxx_want_sample
|
|
|
|
#if !defined(__cpp_lib_boyer_moore_searcher)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_boyer_moore_searcher 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_boyer_moore_searcher)
|
|
# define __cpp_lib_boyer_moore_searcher 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_boyer_moore_searcher) && defined(__glibcxx_want_boyer_moore_searcher) */
|
|
#undef __glibcxx_want_boyer_moore_searcher
|
|
|
|
#if !defined(__cpp_lib_chrono)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_USE_CXX11_ABI && _GLIBCXX_HOSTED
|
|
# define __glibcxx_chrono 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_chrono)
|
|
# define __cpp_lib_chrono 201907L
|
|
# endif
|
|
# elif (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_chrono 201611L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_chrono)
|
|
# define __cpp_lib_chrono 201611L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_chrono) && defined(__glibcxx_want_chrono) */
|
|
#undef __glibcxx_want_chrono
|
|
|
|
#if !defined(__cpp_lib_execution)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_execution 201902L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_execution)
|
|
# define __cpp_lib_execution 201902L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_execution) && defined(__glibcxx_want_execution) */
|
|
#undef __glibcxx_want_execution
|
|
|
|
#if !defined(__cpp_lib_filesystem)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_filesystem 201703L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_filesystem)
|
|
# define __cpp_lib_filesystem 201703L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_filesystem) && defined(__glibcxx_want_filesystem) */
|
|
#undef __glibcxx_want_filesystem
|
|
|
|
#if !defined(__cpp_lib_hypot)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_hypot 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_hypot)
|
|
# define __cpp_lib_hypot 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_hypot) && defined(__glibcxx_want_hypot) */
|
|
#undef __glibcxx_want_hypot
|
|
|
|
#if !defined(__cpp_lib_map_try_emplace)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_map_try_emplace 201411L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_map_try_emplace)
|
|
# define __cpp_lib_map_try_emplace 201411L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_map_try_emplace) && defined(__glibcxx_want_map_try_emplace) */
|
|
#undef __glibcxx_want_map_try_emplace
|
|
|
|
#if !defined(__cpp_lib_math_special_functions)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_math_special_functions 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_math_special_functions)
|
|
# define __cpp_lib_math_special_functions 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_math_special_functions) && defined(__glibcxx_want_math_special_functions) */
|
|
#undef __glibcxx_want_math_special_functions
|
|
|
|
#if !defined(__cpp_lib_memory_resource)
|
|
# if (__cplusplus >= 201703L) && defined(_GLIBCXX_HAS_GTHREADS) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_memory_resource 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_memory_resource)
|
|
# define __cpp_lib_memory_resource 201603L
|
|
# endif
|
|
# elif (__cplusplus >= 201703L) && !defined(_GLIBCXX_HAS_GTHREADS) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_memory_resource 1L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_memory_resource)
|
|
# define __cpp_lib_memory_resource 1L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_memory_resource) && defined(__glibcxx_want_memory_resource) */
|
|
#undef __glibcxx_want_memory_resource
|
|
|
|
#if !defined(__cpp_lib_node_extract)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_node_extract 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_node_extract)
|
|
# define __cpp_lib_node_extract 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_node_extract) && defined(__glibcxx_want_node_extract) */
|
|
#undef __glibcxx_want_node_extract
|
|
|
|
#if !defined(__cpp_lib_parallel_algorithm)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_parallel_algorithm 201603L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_parallel_algorithm)
|
|
# define __cpp_lib_parallel_algorithm 201603L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_parallel_algorithm) && defined(__glibcxx_want_parallel_algorithm) */
|
|
#undef __glibcxx_want_parallel_algorithm
|
|
|
|
#if !defined(__cpp_lib_scoped_lock)
|
|
# if (__cplusplus >= 201703L) && defined(_GLIBCXX_HAS_GTHREADS) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_scoped_lock 201703L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_scoped_lock)
|
|
# define __cpp_lib_scoped_lock 201703L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_scoped_lock) && defined(__glibcxx_want_scoped_lock) */
|
|
#undef __glibcxx_want_scoped_lock
|
|
|
|
#if !defined(__cpp_lib_shared_mutex)
|
|
# if (__cplusplus >= 201703L) && defined(_GLIBCXX_HAS_GTHREADS) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_shared_mutex 201505L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_shared_mutex)
|
|
# define __cpp_lib_shared_mutex 201505L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_shared_mutex) && defined(__glibcxx_want_shared_mutex) */
|
|
#undef __glibcxx_want_shared_mutex
|
|
|
|
#if !defined(__cpp_lib_shared_ptr_weak_type)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_shared_ptr_weak_type 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_shared_ptr_weak_type)
|
|
# define __cpp_lib_shared_ptr_weak_type 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_shared_ptr_weak_type) && defined(__glibcxx_want_shared_ptr_weak_type) */
|
|
#undef __glibcxx_want_shared_ptr_weak_type
|
|
|
|
#if !defined(__cpp_lib_string_view)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_string_view 201803L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_string_view)
|
|
# define __cpp_lib_string_view 201803L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_string_view) && defined(__glibcxx_want_string_view) */
|
|
#undef __glibcxx_want_string_view
|
|
|
|
#if !defined(__cpp_lib_unordered_map_try_emplace)
|
|
# if (__cplusplus >= 201703L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_unordered_map_try_emplace 201411L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_unordered_map_try_emplace)
|
|
# define __cpp_lib_unordered_map_try_emplace 201411L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_unordered_map_try_emplace) && defined(__glibcxx_want_unordered_map_try_emplace) */
|
|
#undef __glibcxx_want_unordered_map_try_emplace
|
|
|
|
#if !defined(__cpp_lib_assume_aligned)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_assume_aligned 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_assume_aligned)
|
|
# define __cpp_lib_assume_aligned 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_assume_aligned) && defined(__glibcxx_want_assume_aligned) */
|
|
#undef __glibcxx_want_assume_aligned
|
|
|
|
#if !defined(__cpp_lib_atomic_flag_test)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_atomic_flag_test 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_atomic_flag_test)
|
|
# define __cpp_lib_atomic_flag_test 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_atomic_flag_test) && defined(__glibcxx_want_atomic_flag_test) */
|
|
#undef __glibcxx_want_atomic_flag_test
|
|
|
|
#if !defined(__cpp_lib_atomic_float)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_atomic_float 201711L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_atomic_float)
|
|
# define __cpp_lib_atomic_float 201711L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_atomic_float) && defined(__glibcxx_want_atomic_float) */
|
|
#undef __glibcxx_want_atomic_float
|
|
|
|
#if !defined(__cpp_lib_atomic_lock_free_type_aliases)
|
|
# if (__cplusplus >= 202002L) && ((__GCC_ATOMIC_INT_LOCK_FREE | __GCC_ATOMIC_LONG_LOCK_FREE | __GCC_ATOMIC_CHAR_LOCK_FREE) & 2)
|
|
# define __glibcxx_atomic_lock_free_type_aliases 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_atomic_lock_free_type_aliases)
|
|
# define __cpp_lib_atomic_lock_free_type_aliases 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_atomic_lock_free_type_aliases) && defined(__glibcxx_want_atomic_lock_free_type_aliases) */
|
|
#undef __glibcxx_want_atomic_lock_free_type_aliases
|
|
|
|
#if !defined(__cpp_lib_atomic_ref)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_atomic_ref 201806L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_atomic_ref)
|
|
# define __cpp_lib_atomic_ref 201806L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_atomic_ref) && defined(__glibcxx_want_atomic_ref) */
|
|
#undef __glibcxx_want_atomic_ref
|
|
|
|
#if !defined(__cpp_lib_atomic_value_initialization)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_atomic_value_initialization 201911L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_atomic_value_initialization)
|
|
# define __cpp_lib_atomic_value_initialization 201911L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_atomic_value_initialization) && defined(__glibcxx_want_atomic_value_initialization) */
|
|
#undef __glibcxx_want_atomic_value_initialization
|
|
|
|
#if !defined(__cpp_lib_bind_front)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_bind_front 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_bind_front)
|
|
# define __cpp_lib_bind_front 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_bind_front) && defined(__glibcxx_want_bind_front) */
|
|
#undef __glibcxx_want_bind_front
|
|
|
|
#if !defined(__cpp_lib_bind_back)
|
|
# if (__cplusplus >= 202100L) && (__cpp_explicit_this_parameter)
|
|
# define __glibcxx_bind_back 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_bind_back)
|
|
# define __cpp_lib_bind_back 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_bind_back) && defined(__glibcxx_want_bind_back) */
|
|
#undef __glibcxx_want_bind_back
|
|
|
|
#if !defined(__cpp_lib_starts_ends_with)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_starts_ends_with 201711L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_starts_ends_with)
|
|
# define __cpp_lib_starts_ends_with 201711L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_starts_ends_with) && defined(__glibcxx_want_starts_ends_with) */
|
|
#undef __glibcxx_want_starts_ends_with
|
|
|
|
#if !defined(__cpp_lib_bit_cast)
|
|
# if (__cplusplus >= 202002L) && (__has_builtin(__builtin_bit_cast))
|
|
# define __glibcxx_bit_cast 201806L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_bit_cast)
|
|
# define __cpp_lib_bit_cast 201806L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_bit_cast) && defined(__glibcxx_want_bit_cast) */
|
|
#undef __glibcxx_want_bit_cast
|
|
|
|
#if !defined(__cpp_lib_bitops)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_bitops 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_bitops)
|
|
# define __cpp_lib_bitops 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_bitops) && defined(__glibcxx_want_bitops) */
|
|
#undef __glibcxx_want_bitops
|
|
|
|
#if !defined(__cpp_lib_bounded_array_traits)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_bounded_array_traits 201902L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_bounded_array_traits)
|
|
# define __cpp_lib_bounded_array_traits 201902L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_bounded_array_traits) && defined(__glibcxx_want_bounded_array_traits) */
|
|
#undef __glibcxx_want_bounded_array_traits
|
|
|
|
#if !defined(__cpp_lib_concepts)
|
|
# if (__cplusplus >= 202002L) && (__cpp_concepts >= 201907L)
|
|
# define __glibcxx_concepts 202002L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_concepts)
|
|
# define __cpp_lib_concepts 202002L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_concepts) && defined(__glibcxx_want_concepts) */
|
|
#undef __glibcxx_want_concepts
|
|
|
|
#if !defined(__cpp_lib_optional)
|
|
# if (__cplusplus >= 202100L) && (__glibcxx_concepts)
|
|
# define __glibcxx_optional 202110L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_optional)
|
|
# define __cpp_lib_optional 202110L
|
|
# endif
|
|
# elif (__cplusplus >= 202002L)
|
|
# define __glibcxx_optional 202106L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_optional)
|
|
# define __cpp_lib_optional 202106L
|
|
# endif
|
|
# elif (__cplusplus >= 201703L)
|
|
# define __glibcxx_optional 201606L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_optional)
|
|
# define __cpp_lib_optional 201606L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_optional) && defined(__glibcxx_want_optional) */
|
|
#undef __glibcxx_want_optional
|
|
|
|
#if !defined(__cpp_lib_destroying_delete)
|
|
# if (__cplusplus >= 202002L) && (__cpp_impl_destroying_delete)
|
|
# define __glibcxx_destroying_delete 201806L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_destroying_delete)
|
|
# define __cpp_lib_destroying_delete 201806L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_destroying_delete) && defined(__glibcxx_want_destroying_delete) */
|
|
#undef __glibcxx_want_destroying_delete
|
|
|
|
#if !defined(__cpp_lib_constexpr_string_view)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_constexpr_string_view 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_string_view)
|
|
# define __cpp_lib_constexpr_string_view 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_string_view) && defined(__glibcxx_want_constexpr_string_view) */
|
|
#undef __glibcxx_want_constexpr_string_view
|
|
|
|
#if !defined(__cpp_lib_endian)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_endian 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_endian)
|
|
# define __cpp_lib_endian 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_endian) && defined(__glibcxx_want_endian) */
|
|
#undef __glibcxx_want_endian
|
|
|
|
#if !defined(__cpp_lib_int_pow2)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_int_pow2 202002L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_int_pow2)
|
|
# define __cpp_lib_int_pow2 202002L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_int_pow2) && defined(__glibcxx_want_int_pow2) */
|
|
#undef __glibcxx_want_int_pow2
|
|
|
|
#if !defined(__cpp_lib_integer_comparison_functions)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_integer_comparison_functions 202002L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_integer_comparison_functions)
|
|
# define __cpp_lib_integer_comparison_functions 202002L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_integer_comparison_functions) && defined(__glibcxx_want_integer_comparison_functions) */
|
|
#undef __glibcxx_want_integer_comparison_functions
|
|
|
|
#if !defined(__cpp_lib_is_constant_evaluated)
|
|
# if (__cplusplus >= 202002L) && (defined(_GLIBCXX_HAVE_IS_CONSTANT_EVALUATED))
|
|
# define __glibcxx_is_constant_evaluated 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_constant_evaluated)
|
|
# define __cpp_lib_is_constant_evaluated 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_constant_evaluated) && defined(__glibcxx_want_is_constant_evaluated) */
|
|
#undef __glibcxx_want_is_constant_evaluated
|
|
|
|
#if !defined(__cpp_lib_constexpr_char_traits)
|
|
# if (__cplusplus >= 202002L) && (defined(__glibcxx_is_constant_evaluated))
|
|
# define __glibcxx_constexpr_char_traits 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_char_traits)
|
|
# define __cpp_lib_constexpr_char_traits 201811L
|
|
# endif
|
|
# elif (__cplusplus >= 201703L) && (_GLIBCXX_HAVE_IS_CONSTANT_EVALUATED)
|
|
# define __glibcxx_constexpr_char_traits 201611L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_char_traits)
|
|
# define __cpp_lib_constexpr_char_traits 201611L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_char_traits) && defined(__glibcxx_want_constexpr_char_traits) */
|
|
#undef __glibcxx_want_constexpr_char_traits
|
|
|
|
#if !defined(__cpp_lib_is_layout_compatible)
|
|
# if (__cplusplus >= 202002L) && (__has_builtin(__is_layout_compatible) && __has_builtin(__builtin_is_corresponding_member))
|
|
# define __glibcxx_is_layout_compatible 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_layout_compatible)
|
|
# define __cpp_lib_is_layout_compatible 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_layout_compatible) && defined(__glibcxx_want_is_layout_compatible) */
|
|
#undef __glibcxx_want_is_layout_compatible
|
|
|
|
#if !defined(__cpp_lib_is_nothrow_convertible)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_is_nothrow_convertible 201806L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_nothrow_convertible)
|
|
# define __cpp_lib_is_nothrow_convertible 201806L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_nothrow_convertible) && defined(__glibcxx_want_is_nothrow_convertible) */
|
|
#undef __glibcxx_want_is_nothrow_convertible
|
|
|
|
#if !defined(__cpp_lib_is_pointer_interconvertible)
|
|
# if (__cplusplus >= 202002L) && (__has_builtin(__is_pointer_interconvertible_base_of) && __has_builtin(__builtin_is_pointer_interconvertible_with_class))
|
|
# define __glibcxx_is_pointer_interconvertible 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_pointer_interconvertible)
|
|
# define __cpp_lib_is_pointer_interconvertible 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_pointer_interconvertible) && defined(__glibcxx_want_is_pointer_interconvertible) */
|
|
#undef __glibcxx_want_is_pointer_interconvertible
|
|
|
|
#if !defined(__cpp_lib_math_constants)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_math_constants 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_math_constants)
|
|
# define __cpp_lib_math_constants 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_math_constants) && defined(__glibcxx_want_math_constants) */
|
|
#undef __glibcxx_want_math_constants
|
|
|
|
#if !defined(__cpp_lib_make_obj_using_allocator)
|
|
# if (__cplusplus >= 202002L) && (__cpp_concepts)
|
|
# define __glibcxx_make_obj_using_allocator 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_make_obj_using_allocator)
|
|
# define __cpp_lib_make_obj_using_allocator 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_make_obj_using_allocator) && defined(__glibcxx_want_make_obj_using_allocator) */
|
|
#undef __glibcxx_want_make_obj_using_allocator
|
|
|
|
#if !defined(__cpp_lib_remove_cvref)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_remove_cvref 201711L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_remove_cvref)
|
|
# define __cpp_lib_remove_cvref 201711L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_remove_cvref) && defined(__glibcxx_want_remove_cvref) */
|
|
#undef __glibcxx_want_remove_cvref
|
|
|
|
#if !defined(__cpp_lib_source_location)
|
|
# if (__cplusplus >= 202002L) && (__has_builtin(__builtin_source_location))
|
|
# define __glibcxx_source_location 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_source_location)
|
|
# define __cpp_lib_source_location 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_source_location) && defined(__glibcxx_want_source_location) */
|
|
#undef __glibcxx_want_source_location
|
|
|
|
#if !defined(__cpp_lib_span)
|
|
# if (__cplusplus > 202302L) && (__glibcxx_concepts)
|
|
# define __glibcxx_span 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_span)
|
|
# define __cpp_lib_span 202311L
|
|
# endif
|
|
# elif (__cplusplus >= 202002L) && (__glibcxx_concepts)
|
|
# define __glibcxx_span 202002L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_span)
|
|
# define __cpp_lib_span 202002L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_span) && defined(__glibcxx_want_span) */
|
|
#undef __glibcxx_want_span
|
|
|
|
#if !defined(__cpp_lib_ssize)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_ssize 201902L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ssize)
|
|
# define __cpp_lib_ssize 201902L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ssize) && defined(__glibcxx_want_ssize) */
|
|
#undef __glibcxx_want_ssize
|
|
|
|
#if !defined(__cpp_lib_three_way_comparison)
|
|
# if (__cplusplus >= 202002L) && (__cpp_impl_three_way_comparison >= 201907L && __glibcxx_concepts)
|
|
# define __glibcxx_three_way_comparison 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_three_way_comparison)
|
|
# define __cpp_lib_three_way_comparison 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_three_way_comparison) && defined(__glibcxx_want_three_way_comparison) */
|
|
#undef __glibcxx_want_three_way_comparison
|
|
|
|
#if !defined(__cpp_lib_to_address)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_to_address 201711L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_to_address)
|
|
# define __cpp_lib_to_address 201711L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_to_address) && defined(__glibcxx_want_to_address) */
|
|
#undef __glibcxx_want_to_address
|
|
|
|
#if !defined(__cpp_lib_to_array)
|
|
# if (__cplusplus >= 202002L) && (__cpp_generic_lambdas >= 201707L)
|
|
# define __glibcxx_to_array 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_to_array)
|
|
# define __cpp_lib_to_array 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_to_array) && defined(__glibcxx_want_to_array) */
|
|
#undef __glibcxx_want_to_array
|
|
|
|
#if !defined(__cpp_lib_type_identity)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_type_identity 201806L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_type_identity)
|
|
# define __cpp_lib_type_identity 201806L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_type_identity) && defined(__glibcxx_want_type_identity) */
|
|
#undef __glibcxx_want_type_identity
|
|
|
|
#if !defined(__cpp_lib_unwrap_ref)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_unwrap_ref 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_unwrap_ref)
|
|
# define __cpp_lib_unwrap_ref 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_unwrap_ref) && defined(__glibcxx_want_unwrap_ref) */
|
|
#undef __glibcxx_want_unwrap_ref
|
|
|
|
#if !defined(__cpp_lib_constexpr_iterator)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_constexpr_iterator 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_iterator)
|
|
# define __cpp_lib_constexpr_iterator 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_iterator) && defined(__glibcxx_want_constexpr_iterator) */
|
|
#undef __glibcxx_want_constexpr_iterator
|
|
|
|
#if !defined(__cpp_lib_interpolate)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_interpolate 201902L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_interpolate)
|
|
# define __cpp_lib_interpolate 201902L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_interpolate) && defined(__glibcxx_want_interpolate) */
|
|
#undef __glibcxx_want_interpolate
|
|
|
|
#if !defined(__cpp_lib_constexpr_utility)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_constexpr_utility 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_utility)
|
|
# define __cpp_lib_constexpr_utility 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_utility) && defined(__glibcxx_want_constexpr_utility) */
|
|
#undef __glibcxx_want_constexpr_utility
|
|
|
|
#if !defined(__cpp_lib_shift)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_shift 201806L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_shift)
|
|
# define __cpp_lib_shift 201806L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_shift) && defined(__glibcxx_want_shift) */
|
|
#undef __glibcxx_want_shift
|
|
|
|
#if !defined(__cpp_lib_ranges)
|
|
# if (__cplusplus >= 202100L) && (__glibcxx_concepts)
|
|
# define __glibcxx_ranges 202211L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges)
|
|
# define __cpp_lib_ranges 202211L
|
|
# endif
|
|
# elif (__cplusplus >= 202002L) && (__glibcxx_concepts)
|
|
# define __glibcxx_ranges 202110L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges)
|
|
# define __cpp_lib_ranges 202110L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges) && defined(__glibcxx_want_ranges) */
|
|
#undef __glibcxx_want_ranges
|
|
|
|
#if !defined(__cpp_lib_constexpr_numeric)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_constexpr_numeric 201911L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_numeric)
|
|
# define __cpp_lib_constexpr_numeric 201911L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_numeric) && defined(__glibcxx_want_constexpr_numeric) */
|
|
#undef __glibcxx_want_constexpr_numeric
|
|
|
|
#if !defined(__cpp_lib_constexpr_functional)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_constexpr_functional 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_functional)
|
|
# define __cpp_lib_constexpr_functional 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_functional) && defined(__glibcxx_want_constexpr_functional) */
|
|
#undef __glibcxx_want_constexpr_functional
|
|
|
|
#if !defined(__cpp_lib_constexpr_algorithms)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_constexpr_algorithms 201806L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_algorithms)
|
|
# define __cpp_lib_constexpr_algorithms 201806L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_algorithms) && defined(__glibcxx_want_constexpr_algorithms) */
|
|
#undef __glibcxx_want_constexpr_algorithms
|
|
|
|
#if !defined(__cpp_lib_constexpr_tuple)
|
|
# if (__cplusplus >= 202002L)
|
|
# define __glibcxx_constexpr_tuple 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_tuple)
|
|
# define __cpp_lib_constexpr_tuple 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_tuple) && defined(__glibcxx_want_constexpr_tuple) */
|
|
#undef __glibcxx_want_constexpr_tuple
|
|
|
|
#if !defined(__cpp_lib_constexpr_memory)
|
|
# if (__cplusplus >= 202100L) && (__cpp_constexpr_dynamic_alloc)
|
|
# define __glibcxx_constexpr_memory 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_memory)
|
|
# define __cpp_lib_constexpr_memory 202202L
|
|
# endif
|
|
# elif (__cplusplus >= 202002L)
|
|
# define __glibcxx_constexpr_memory 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_memory)
|
|
# define __cpp_lib_constexpr_memory 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_memory) && defined(__glibcxx_want_constexpr_memory) */
|
|
#undef __glibcxx_want_constexpr_memory
|
|
|
|
#if !defined(__cpp_lib_atomic_shared_ptr)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_atomic_shared_ptr 201711L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_atomic_shared_ptr)
|
|
# define __cpp_lib_atomic_shared_ptr 201711L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_atomic_shared_ptr) && defined(__glibcxx_want_atomic_shared_ptr) */
|
|
#undef __glibcxx_want_atomic_shared_ptr
|
|
|
|
#if !defined(__cpp_lib_atomic_wait)
|
|
# if (__cplusplus >= 202002L) && defined(_GLIBCXX_HAS_GTHREADS) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_atomic_wait 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_atomic_wait)
|
|
# define __cpp_lib_atomic_wait 201907L
|
|
# endif
|
|
# elif (__cplusplus >= 202002L) && !defined(_GLIBCXX_HAS_GTHREADS) && _GLIBCXX_HOSTED && (defined(_GLIBCXX_HAVE_LINUX_FUTEX))
|
|
# define __glibcxx_atomic_wait 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_atomic_wait)
|
|
# define __cpp_lib_atomic_wait 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_atomic_wait) && defined(__glibcxx_want_atomic_wait) */
|
|
#undef __glibcxx_want_atomic_wait
|
|
|
|
#if !defined(__cpp_lib_barrier)
|
|
# if (__cplusplus >= 202002L) && (__cpp_aligned_new && __glibcxx_atomic_wait)
|
|
# define __glibcxx_barrier 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_barrier)
|
|
# define __cpp_lib_barrier 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_barrier) && defined(__glibcxx_want_barrier) */
|
|
#undef __glibcxx_want_barrier
|
|
|
|
#if !defined(__cpp_lib_format)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_format 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_format)
|
|
# define __cpp_lib_format 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_format) && defined(__glibcxx_want_format) */
|
|
#undef __glibcxx_want_format
|
|
|
|
#if !defined(__cpp_lib_format_uchar)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_format_uchar 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_format_uchar)
|
|
# define __cpp_lib_format_uchar 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_format_uchar) && defined(__glibcxx_want_format_uchar) */
|
|
#undef __glibcxx_want_format_uchar
|
|
|
|
#if !defined(__cpp_lib_constexpr_complex)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_constexpr_complex 201711L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_complex)
|
|
# define __cpp_lib_constexpr_complex 201711L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_complex) && defined(__glibcxx_want_constexpr_complex) */
|
|
#undef __glibcxx_want_constexpr_complex
|
|
|
|
#if !defined(__cpp_lib_constexpr_dynamic_alloc)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_constexpr_dynamic_alloc 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_dynamic_alloc)
|
|
# define __cpp_lib_constexpr_dynamic_alloc 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_dynamic_alloc) && defined(__glibcxx_want_constexpr_dynamic_alloc) */
|
|
#undef __glibcxx_want_constexpr_dynamic_alloc
|
|
|
|
#if !defined(__cpp_lib_constexpr_string)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_USE_CXX11_ABI && _GLIBCXX_HOSTED && (defined(__glibcxx_is_constant_evaluated))
|
|
# define __glibcxx_constexpr_string 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_string)
|
|
# define __cpp_lib_constexpr_string 201907L
|
|
# endif
|
|
# elif (__cplusplus >= 202002L) && !_GLIBCXX_USE_CXX11_ABI && _GLIBCXX_HOSTED && (defined(__glibcxx_is_constant_evaluated))
|
|
# define __glibcxx_constexpr_string 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_string)
|
|
# define __cpp_lib_constexpr_string 201811L
|
|
# endif
|
|
# elif (__cplusplus >= 201703L) && _GLIBCXX_HOSTED && (_GLIBCXX_HAVE_IS_CONSTANT_EVALUATED)
|
|
# define __glibcxx_constexpr_string 201611L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_string)
|
|
# define __cpp_lib_constexpr_string 201611L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_string) && defined(__glibcxx_want_constexpr_string) */
|
|
#undef __glibcxx_want_constexpr_string
|
|
|
|
#if !defined(__cpp_lib_constexpr_vector)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_constexpr_vector 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_vector)
|
|
# define __cpp_lib_constexpr_vector 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_vector) && defined(__glibcxx_want_constexpr_vector) */
|
|
#undef __glibcxx_want_constexpr_vector
|
|
|
|
#if !defined(__cpp_lib_constrained_equality)
|
|
# if (__cplusplus >= 202002L) && (__glibcxx_three_way_comparison)
|
|
# define __glibcxx_constrained_equality 202403L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constrained_equality)
|
|
# define __cpp_lib_constrained_equality 202403L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constrained_equality) && defined(__glibcxx_want_constrained_equality) */
|
|
#undef __glibcxx_want_constrained_equality
|
|
|
|
#if !defined(__cpp_lib_erase_if)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_erase_if 202002L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_erase_if)
|
|
# define __cpp_lib_erase_if 202002L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_erase_if) && defined(__glibcxx_want_erase_if) */
|
|
#undef __glibcxx_want_erase_if
|
|
|
|
#if !defined(__cpp_lib_generic_unordered_lookup)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_generic_unordered_lookup 201811L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_generic_unordered_lookup)
|
|
# define __cpp_lib_generic_unordered_lookup 201811L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_generic_unordered_lookup) && defined(__glibcxx_want_generic_unordered_lookup) */
|
|
#undef __glibcxx_want_generic_unordered_lookup
|
|
|
|
#if !defined(__cpp_lib_jthread)
|
|
# if (__cplusplus >= 202002L) && defined(_GLIBCXX_HAS_GTHREADS) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_jthread 201911L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_jthread)
|
|
# define __cpp_lib_jthread 201911L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_jthread) && defined(__glibcxx_want_jthread) */
|
|
#undef __glibcxx_want_jthread
|
|
|
|
#if !defined(__cpp_lib_latch)
|
|
# if (__cplusplus >= 202002L) && (__glibcxx_atomic_wait)
|
|
# define __glibcxx_latch 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_latch)
|
|
# define __cpp_lib_latch 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_latch) && defined(__glibcxx_want_latch) */
|
|
#undef __glibcxx_want_latch
|
|
|
|
#if !defined(__cpp_lib_list_remove_return_type)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_list_remove_return_type 201806L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_list_remove_return_type)
|
|
# define __cpp_lib_list_remove_return_type 201806L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_list_remove_return_type) && defined(__glibcxx_want_list_remove_return_type) */
|
|
#undef __glibcxx_want_list_remove_return_type
|
|
|
|
#if !defined(__cpp_lib_polymorphic_allocator)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_polymorphic_allocator 201902L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_polymorphic_allocator)
|
|
# define __cpp_lib_polymorphic_allocator 201902L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_polymorphic_allocator) && defined(__glibcxx_want_polymorphic_allocator) */
|
|
#undef __glibcxx_want_polymorphic_allocator
|
|
|
|
#if !defined(__cpp_lib_move_iterator_concept)
|
|
# if (__cplusplus >= 202002L) && (__glibcxx_concepts)
|
|
# define __glibcxx_move_iterator_concept 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_move_iterator_concept)
|
|
# define __cpp_lib_move_iterator_concept 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_move_iterator_concept) && defined(__glibcxx_want_move_iterator_concept) */
|
|
#undef __glibcxx_want_move_iterator_concept
|
|
|
|
#if !defined(__cpp_lib_semaphore)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED && (__glibcxx_atomic_wait || _GLIBCXX_HAVE_POSIX_SEMAPHORE)
|
|
# define __glibcxx_semaphore 201907L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_semaphore)
|
|
# define __cpp_lib_semaphore 201907L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_semaphore) && defined(__glibcxx_want_semaphore) */
|
|
#undef __glibcxx_want_semaphore
|
|
|
|
#if !defined(__cpp_lib_smart_ptr_for_overwrite)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_smart_ptr_for_overwrite 202002L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_smart_ptr_for_overwrite)
|
|
# define __cpp_lib_smart_ptr_for_overwrite 202002L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_smart_ptr_for_overwrite) && defined(__glibcxx_want_smart_ptr_for_overwrite) */
|
|
#undef __glibcxx_want_smart_ptr_for_overwrite
|
|
|
|
#if !defined(__cpp_lib_syncbuf)
|
|
# if (__cplusplus >= 202002L) && _GLIBCXX_USE_CXX11_ABI && _GLIBCXX_HOSTED
|
|
# define __glibcxx_syncbuf 201803L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_syncbuf)
|
|
# define __cpp_lib_syncbuf 201803L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_syncbuf) && defined(__glibcxx_want_syncbuf) */
|
|
#undef __glibcxx_want_syncbuf
|
|
|
|
#if !defined(__cpp_lib_byteswap)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_byteswap 202110L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_byteswap)
|
|
# define __cpp_lib_byteswap 202110L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_byteswap) && defined(__glibcxx_want_byteswap) */
|
|
#undef __glibcxx_want_byteswap
|
|
|
|
#if !defined(__cpp_lib_constexpr_charconv)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_constexpr_charconv 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_charconv)
|
|
# define __cpp_lib_constexpr_charconv 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_charconv) && defined(__glibcxx_want_constexpr_charconv) */
|
|
#undef __glibcxx_want_constexpr_charconv
|
|
|
|
#if !defined(__cpp_lib_constexpr_typeinfo)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_constexpr_typeinfo 202106L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_typeinfo)
|
|
# define __cpp_lib_constexpr_typeinfo 202106L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_typeinfo) && defined(__glibcxx_want_constexpr_typeinfo) */
|
|
#undef __glibcxx_want_constexpr_typeinfo
|
|
|
|
#if !defined(__cpp_lib_expected)
|
|
# if (__cplusplus >= 202100L) && (__cpp_concepts >= 202002L)
|
|
# define __glibcxx_expected 202211L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_expected)
|
|
# define __cpp_lib_expected 202211L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_expected) && defined(__glibcxx_want_expected) */
|
|
#undef __glibcxx_want_expected
|
|
|
|
#if !defined(__cpp_lib_freestanding_algorithm)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_freestanding_algorithm 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_freestanding_algorithm)
|
|
# define __cpp_lib_freestanding_algorithm 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_freestanding_algorithm) && defined(__glibcxx_want_freestanding_algorithm) */
|
|
#undef __glibcxx_want_freestanding_algorithm
|
|
|
|
#if !defined(__cpp_lib_freestanding_array)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_freestanding_array 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_freestanding_array)
|
|
# define __cpp_lib_freestanding_array 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_freestanding_array) && defined(__glibcxx_want_freestanding_array) */
|
|
#undef __glibcxx_want_freestanding_array
|
|
|
|
#if !defined(__cpp_lib_freestanding_cstring)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_freestanding_cstring 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_freestanding_cstring)
|
|
# define __cpp_lib_freestanding_cstring 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_freestanding_cstring) && defined(__glibcxx_want_freestanding_cstring) */
|
|
#undef __glibcxx_want_freestanding_cstring
|
|
|
|
#if !defined(__cpp_lib_freestanding_expected)
|
|
# if (__cplusplus >= 202100L) && (__cpp_lib_expected)
|
|
# define __glibcxx_freestanding_expected 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_freestanding_expected)
|
|
# define __cpp_lib_freestanding_expected 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_freestanding_expected) && defined(__glibcxx_want_freestanding_expected) */
|
|
#undef __glibcxx_want_freestanding_expected
|
|
|
|
#if !defined(__cpp_lib_freestanding_optional)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_freestanding_optional 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_freestanding_optional)
|
|
# define __cpp_lib_freestanding_optional 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_freestanding_optional) && defined(__glibcxx_want_freestanding_optional) */
|
|
#undef __glibcxx_want_freestanding_optional
|
|
|
|
#if !defined(__cpp_lib_freestanding_string_view)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_freestanding_string_view 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_freestanding_string_view)
|
|
# define __cpp_lib_freestanding_string_view 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_freestanding_string_view) && defined(__glibcxx_want_freestanding_string_view) */
|
|
#undef __glibcxx_want_freestanding_string_view
|
|
|
|
#if !defined(__cpp_lib_freestanding_variant)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_freestanding_variant 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_freestanding_variant)
|
|
# define __cpp_lib_freestanding_variant 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_freestanding_variant) && defined(__glibcxx_want_freestanding_variant) */
|
|
#undef __glibcxx_want_freestanding_variant
|
|
|
|
#if !defined(__cpp_lib_invoke_r)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_invoke_r 202106L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_invoke_r)
|
|
# define __cpp_lib_invoke_r 202106L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_invoke_r) && defined(__glibcxx_want_invoke_r) */
|
|
#undef __glibcxx_want_invoke_r
|
|
|
|
#if !defined(__cpp_lib_is_scoped_enum)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_is_scoped_enum 202011L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_is_scoped_enum)
|
|
# define __cpp_lib_is_scoped_enum 202011L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_is_scoped_enum) && defined(__glibcxx_want_is_scoped_enum) */
|
|
#undef __glibcxx_want_is_scoped_enum
|
|
|
|
#if !defined(__cpp_lib_reference_from_temporary)
|
|
# if (__cplusplus >= 202100L) && (__has_builtin(__reference_constructs_from_temporary) && __has_builtin(__reference_converts_from_temporary))
|
|
# define __glibcxx_reference_from_temporary 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_reference_from_temporary)
|
|
# define __cpp_lib_reference_from_temporary 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_reference_from_temporary) && defined(__glibcxx_want_reference_from_temporary) */
|
|
#undef __glibcxx_want_reference_from_temporary
|
|
|
|
#if !defined(__cpp_lib_ranges_to_container)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_ranges_to_container 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_to_container)
|
|
# define __cpp_lib_ranges_to_container 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_to_container) && defined(__glibcxx_want_ranges_to_container) */
|
|
#undef __glibcxx_want_ranges_to_container
|
|
|
|
#if !defined(__cpp_lib_ranges_zip)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_zip 202110L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_zip)
|
|
# define __cpp_lib_ranges_zip 202110L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_zip) && defined(__glibcxx_want_ranges_zip) */
|
|
#undef __glibcxx_want_ranges_zip
|
|
|
|
#if !defined(__cpp_lib_ranges_chunk)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_chunk 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_chunk)
|
|
# define __cpp_lib_ranges_chunk 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_chunk) && defined(__glibcxx_want_ranges_chunk) */
|
|
#undef __glibcxx_want_ranges_chunk
|
|
|
|
#if !defined(__cpp_lib_ranges_slide)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_slide 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_slide)
|
|
# define __cpp_lib_ranges_slide 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_slide) && defined(__glibcxx_want_ranges_slide) */
|
|
#undef __glibcxx_want_ranges_slide
|
|
|
|
#if !defined(__cpp_lib_ranges_chunk_by)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_chunk_by 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_chunk_by)
|
|
# define __cpp_lib_ranges_chunk_by 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_chunk_by) && defined(__glibcxx_want_ranges_chunk_by) */
|
|
#undef __glibcxx_want_ranges_chunk_by
|
|
|
|
#if !defined(__cpp_lib_ranges_join_with)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_join_with 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_join_with)
|
|
# define __cpp_lib_ranges_join_with 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_join_with) && defined(__glibcxx_want_ranges_join_with) */
|
|
#undef __glibcxx_want_ranges_join_with
|
|
|
|
#if !defined(__cpp_lib_ranges_repeat)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_repeat 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_repeat)
|
|
# define __cpp_lib_ranges_repeat 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_repeat) && defined(__glibcxx_want_ranges_repeat) */
|
|
#undef __glibcxx_want_ranges_repeat
|
|
|
|
#if !defined(__cpp_lib_ranges_stride)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_stride 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_stride)
|
|
# define __cpp_lib_ranges_stride 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_stride) && defined(__glibcxx_want_ranges_stride) */
|
|
#undef __glibcxx_want_ranges_stride
|
|
|
|
#if !defined(__cpp_lib_ranges_cartesian_product)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_cartesian_product 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_cartesian_product)
|
|
# define __cpp_lib_ranges_cartesian_product 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_cartesian_product) && defined(__glibcxx_want_ranges_cartesian_product) */
|
|
#undef __glibcxx_want_ranges_cartesian_product
|
|
|
|
#if !defined(__cpp_lib_ranges_as_rvalue)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_as_rvalue 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_as_rvalue)
|
|
# define __cpp_lib_ranges_as_rvalue 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_as_rvalue) && defined(__glibcxx_want_ranges_as_rvalue) */
|
|
#undef __glibcxx_want_ranges_as_rvalue
|
|
|
|
#if !defined(__cpp_lib_ranges_as_const)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_as_const 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_as_const)
|
|
# define __cpp_lib_ranges_as_const 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_as_const) && defined(__glibcxx_want_ranges_as_const) */
|
|
#undef __glibcxx_want_ranges_as_const
|
|
|
|
#if !defined(__cpp_lib_ranges_enumerate)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_enumerate 202302L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_enumerate)
|
|
# define __cpp_lib_ranges_enumerate 202302L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_enumerate) && defined(__glibcxx_want_ranges_enumerate) */
|
|
#undef __glibcxx_want_ranges_enumerate
|
|
|
|
#if !defined(__cpp_lib_ranges_fold)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_fold 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_fold)
|
|
# define __cpp_lib_ranges_fold 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_fold) && defined(__glibcxx_want_ranges_fold) */
|
|
#undef __glibcxx_want_ranges_fold
|
|
|
|
#if !defined(__cpp_lib_ranges_contains)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_contains 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_contains)
|
|
# define __cpp_lib_ranges_contains 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_contains) && defined(__glibcxx_want_ranges_contains) */
|
|
#undef __glibcxx_want_ranges_contains
|
|
|
|
#if !defined(__cpp_lib_ranges_iota)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_iota 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_iota)
|
|
# define __cpp_lib_ranges_iota 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_iota) && defined(__glibcxx_want_ranges_iota) */
|
|
#undef __glibcxx_want_ranges_iota
|
|
|
|
#if !defined(__cpp_lib_ranges_find_last)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_ranges_find_last 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_find_last)
|
|
# define __cpp_lib_ranges_find_last 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_find_last) && defined(__glibcxx_want_ranges_find_last) */
|
|
#undef __glibcxx_want_ranges_find_last
|
|
|
|
#if !defined(__cpp_lib_constexpr_bitset)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED && (__cpp_constexpr_dynamic_alloc)
|
|
# define __glibcxx_constexpr_bitset 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_constexpr_bitset)
|
|
# define __cpp_lib_constexpr_bitset 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_constexpr_bitset) && defined(__glibcxx_want_constexpr_bitset) */
|
|
#undef __glibcxx_want_constexpr_bitset
|
|
|
|
#if !defined(__cpp_lib_stdatomic_h)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_stdatomic_h 202011L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_stdatomic_h)
|
|
# define __cpp_lib_stdatomic_h 202011L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_stdatomic_h) && defined(__glibcxx_want_stdatomic_h) */
|
|
#undef __glibcxx_want_stdatomic_h
|
|
|
|
#if !defined(__cpp_lib_adaptor_iterator_pair_constructor)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_adaptor_iterator_pair_constructor 202106L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_adaptor_iterator_pair_constructor)
|
|
# define __cpp_lib_adaptor_iterator_pair_constructor 202106L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_adaptor_iterator_pair_constructor) && defined(__glibcxx_want_adaptor_iterator_pair_constructor) */
|
|
#undef __glibcxx_want_adaptor_iterator_pair_constructor
|
|
|
|
#if !defined(__cpp_lib_formatters)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_formatters 202302L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_formatters)
|
|
# define __cpp_lib_formatters 202302L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_formatters) && defined(__glibcxx_want_formatters) */
|
|
#undef __glibcxx_want_formatters
|
|
|
|
#if !defined(__cpp_lib_forward_like)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_forward_like 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_forward_like)
|
|
# define __cpp_lib_forward_like 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_forward_like) && defined(__glibcxx_want_forward_like) */
|
|
#undef __glibcxx_want_forward_like
|
|
|
|
#if !defined(__cpp_lib_generator)
|
|
# if (__cplusplus >= 202100L) && (__glibcxx_coroutine && __cpp_sized_deallocation)
|
|
# define __glibcxx_generator 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_generator)
|
|
# define __cpp_lib_generator 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_generator) && defined(__glibcxx_want_generator) */
|
|
#undef __glibcxx_want_generator
|
|
|
|
#if !defined(__cpp_lib_ios_noreplace)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_ios_noreplace 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ios_noreplace)
|
|
# define __cpp_lib_ios_noreplace 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ios_noreplace) && defined(__glibcxx_want_ios_noreplace) */
|
|
#undef __glibcxx_want_ios_noreplace
|
|
|
|
#if !defined(__cpp_lib_move_only_function)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_move_only_function 202110L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_move_only_function)
|
|
# define __cpp_lib_move_only_function 202110L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_move_only_function) && defined(__glibcxx_want_move_only_function) */
|
|
#undef __glibcxx_want_move_only_function
|
|
|
|
#if !defined(__cpp_lib_out_ptr)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_out_ptr 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_out_ptr)
|
|
# define __cpp_lib_out_ptr 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_out_ptr) && defined(__glibcxx_want_out_ptr) */
|
|
#undef __glibcxx_want_out_ptr
|
|
|
|
#if !defined(__cpp_lib_print)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_print 202211L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_print)
|
|
# define __cpp_lib_print 202211L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_print) && defined(__glibcxx_want_print) */
|
|
#undef __glibcxx_want_print
|
|
|
|
#if !defined(__cpp_lib_spanstream)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED && (__glibcxx_span)
|
|
# define __glibcxx_spanstream 202106L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_spanstream)
|
|
# define __cpp_lib_spanstream 202106L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_spanstream) && defined(__glibcxx_want_spanstream) */
|
|
#undef __glibcxx_want_spanstream
|
|
|
|
#if !defined(__cpp_lib_stacktrace)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED && (_GLIBCXX_HAVE_STACKTRACE)
|
|
# define __glibcxx_stacktrace 202011L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_stacktrace)
|
|
# define __cpp_lib_stacktrace 202011L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_stacktrace) && defined(__glibcxx_want_stacktrace) */
|
|
#undef __glibcxx_want_stacktrace
|
|
|
|
#if !defined(__cpp_lib_string_contains)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_string_contains 202011L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_string_contains)
|
|
# define __cpp_lib_string_contains 202011L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_string_contains) && defined(__glibcxx_want_string_contains) */
|
|
#undef __glibcxx_want_string_contains
|
|
|
|
#if !defined(__cpp_lib_string_resize_and_overwrite)
|
|
# if (__cplusplus >= 202100L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_string_resize_and_overwrite 202110L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_string_resize_and_overwrite)
|
|
# define __cpp_lib_string_resize_and_overwrite 202110L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_string_resize_and_overwrite) && defined(__glibcxx_want_string_resize_and_overwrite) */
|
|
#undef __glibcxx_want_string_resize_and_overwrite
|
|
|
|
#if !defined(__cpp_lib_to_underlying)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_to_underlying 202102L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_to_underlying)
|
|
# define __cpp_lib_to_underlying 202102L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_to_underlying) && defined(__glibcxx_want_to_underlying) */
|
|
#undef __glibcxx_want_to_underlying
|
|
|
|
#if !defined(__cpp_lib_tuple_like)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_tuple_like 202207L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_tuple_like)
|
|
# define __cpp_lib_tuple_like 202207L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_tuple_like) && defined(__glibcxx_want_tuple_like) */
|
|
#undef __glibcxx_want_tuple_like
|
|
|
|
#if !defined(__cpp_lib_unreachable)
|
|
# if (__cplusplus >= 202100L)
|
|
# define __glibcxx_unreachable 202202L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_unreachable)
|
|
# define __cpp_lib_unreachable 202202L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_unreachable) && defined(__glibcxx_want_unreachable) */
|
|
#undef __glibcxx_want_unreachable
|
|
|
|
#if !defined(__cpp_lib_fstream_native_handle)
|
|
# if (__cplusplus > 202302L) && _GLIBCXX_HOSTED
|
|
# define __glibcxx_fstream_native_handle 202306L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_fstream_native_handle)
|
|
# define __cpp_lib_fstream_native_handle 202306L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_fstream_native_handle) && defined(__glibcxx_want_fstream_native_handle) */
|
|
#undef __glibcxx_want_fstream_native_handle
|
|
|
|
#if !defined(__cpp_lib_ratio)
|
|
# if (__cplusplus > 202302L)
|
|
# define __glibcxx_ratio 202306L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ratio)
|
|
# define __cpp_lib_ratio 202306L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ratio) && defined(__glibcxx_want_ratio) */
|
|
#undef __glibcxx_want_ratio
|
|
|
|
#if !defined(__cpp_lib_reference_wrapper)
|
|
# if (__cplusplus > 202302L)
|
|
# define __glibcxx_reference_wrapper 202403L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_reference_wrapper)
|
|
# define __cpp_lib_reference_wrapper 202403L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_reference_wrapper) && defined(__glibcxx_want_reference_wrapper) */
|
|
#undef __glibcxx_want_reference_wrapper
|
|
|
|
#if !defined(__cpp_lib_saturation_arithmetic)
|
|
# if (__cplusplus > 202302L)
|
|
# define __glibcxx_saturation_arithmetic 202311L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_saturation_arithmetic)
|
|
# define __cpp_lib_saturation_arithmetic 202311L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_saturation_arithmetic) && defined(__glibcxx_want_saturation_arithmetic) */
|
|
#undef __glibcxx_want_saturation_arithmetic
|
|
|
|
#if !defined(__cpp_lib_text_encoding)
|
|
# if (__cplusplus > 202302L) && _GLIBCXX_HOSTED && (_GLIBCXX_USE_NL_LANGINFO_L)
|
|
# define __glibcxx_text_encoding 202306L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_text_encoding)
|
|
# define __cpp_lib_text_encoding 202306L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_text_encoding) && defined(__glibcxx_want_text_encoding) */
|
|
#undef __glibcxx_want_text_encoding
|
|
|
|
#if !defined(__cpp_lib_to_string)
|
|
# if (__cplusplus > 202302L) && _GLIBCXX_HOSTED && (__glibcxx_to_chars)
|
|
# define __glibcxx_to_string 202306L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_to_string)
|
|
# define __cpp_lib_to_string 202306L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_to_string) && defined(__glibcxx_want_to_string) */
|
|
#undef __glibcxx_want_to_string
|
|
|
|
#if !defined(__cpp_lib_ranges_concat)
|
|
# if (__cplusplus > 202302L)
|
|
# define __glibcxx_ranges_concat 202403L
|
|
# if defined(__glibcxx_want_all) || defined(__glibcxx_want_ranges_concat)
|
|
# define __cpp_lib_ranges_concat 202403L
|
|
# endif
|
|
# endif
|
|
#endif /* !defined(__cpp_lib_ranges_concat) && defined(__glibcxx_want_ranges_concat) */
|
|
#undef __glibcxx_want_ranges_concat
|
|
|
|
#undef __glibcxx_want_all
|