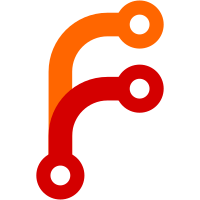
* exec/configure.ac (REENTRANT): Remove option for reentrancy. (PROGRAM_COUNTER, HAVE_SECCOMP): Define register providing the program counter and enable seccomp if its headers are available. * exec/exec.c (write_load_command): Avoid defining unused variable. (exec_0): Remove code specific to REENTRANT configurations. * exec/exec.h (struct exec_tracee) <exec_data, data_size>: New fields for loader instructions and their size. * exec/exec1.c (main): Call exec_init before forking. * exec/mipsel-user.h (ELF_NGREG): Delete definition. (struct mipsel_regs): Reduce number of gregs to 32, but introduce separate fields for special registers. * exec/trace.c (use_seccomp_p): New variable; defile to false if !HAVE_SECCOMP. (remove_tracee): Cease providing for non-reentrant configurations. Release executable data if present. (handle_clone_prepare): Likewise. Resume process with PTRACE_CONT if seccomp-based interception is enabled. (handle_clone, check_signal): Resume processes as above. (handle_exec): Divide into two functions, with only rewriting the system call and generating instructions for the loader remaining in the first, and copying such instructions into the loader's stack removed into a new function, `finish_exec'. (finish_exec): New function. (handle_readlinkat, handle_openat): Abolish non-REENTRANT configurations. (process_system_call): Divide exec system calls into two phases, disambiguated by the value of tracee->waiting_for_syscall. Typo fixes. Accommodate syscall-exit-stops where the signal was initially intercepted by `seccomp_system_call'. (interesting_syscalls): New array. (ARRAYELTS): New macro. (seccomp_system_call, establish_seccomp_filter): New function. (tracing_execve) [HAVE_SECCOMP]: Establish a seccomp filter if this is to be enabled. (after_fork): Provide PTRACE_O_TRACESECCOMP. Resume process with PTRACE_CONT if seccomp-based interception is enabled. (exec_waitpid): Resume process with PTRACE_CONT if seccomp-based interception is enabled. Dispatch stops identifying as PTRACE_EVENT_SECCOMP to `seccomp_system_call'. (exec_init): Establish whether it is possible to enable seccomp.
94 lines
2.3 KiB
C
94 lines
2.3 KiB
C
/* Program execution for Emacs.
|
||
|
||
Copyright (C) 2023-2024 Free Software Foundation, Inc.
|
||
|
||
This file is part of GNU Emacs.
|
||
|
||
GNU Emacs is free software: you can redistribute it and/or modify
|
||
it under the terms of the GNU General Public License as published by
|
||
the Free Software Foundation, either version 3 of the License, or (at
|
||
your option) any later version.
|
||
|
||
GNU Emacs is distributed in the hope that it will be useful,
|
||
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||
GNU General Public License for more details.
|
||
|
||
You should have received a copy of the GNU General Public License
|
||
along with GNU Emacs. If not, see <https://www.gnu.org/licenses/>. */
|
||
|
||
#include <config.h>
|
||
#include <unistd.h>
|
||
#include <stdlib.h>
|
||
#include <stdio.h>
|
||
#include <sys/wait.h>
|
||
|
||
#include "exec.h"
|
||
|
||
/* exec1 is a program which takes another program and its arguments,
|
||
forks, and executes that program, all while tracing it and its
|
||
children to use the program execution mechanism defined in exec.c.
|
||
|
||
This is necessary to bypass security restrictions which prohibit
|
||
Emacs from loading executables from certain directories, by, in
|
||
effect, replacing the executable loader in the Linux kernel. */
|
||
|
||
|
||
|
||
int
|
||
main (int argc, char **argv)
|
||
{
|
||
pid_t pid, pid1;
|
||
extern char **environ;
|
||
int wstatus;
|
||
|
||
/* Provide the file name of the loader. */
|
||
exec_init (argv[1]);
|
||
|
||
pid1 = getpid ();
|
||
pid = fork ();
|
||
|
||
if (!pid)
|
||
{
|
||
/* Set the process group used to the parent. */
|
||
if (setpgid (0, pid1))
|
||
perror ("setpgid");
|
||
|
||
tracing_execve (argv[2], argv + 2, environ);
|
||
|
||
/* An error occurred. Exit with failure. */
|
||
exit (127);
|
||
}
|
||
else
|
||
{
|
||
if (after_fork (pid))
|
||
exit (127);
|
||
|
||
/* Start waiting for the process to exit. */
|
||
|
||
while (true)
|
||
{
|
||
pid1 = exec_waitpid (-1, &wstatus, 0);
|
||
|
||
/* If the child process exits normally, exit with its status
|
||
code. If not, raise the signal that caused it to
|
||
exit. */
|
||
|
||
if (pid == pid1)
|
||
{
|
||
if (WIFEXITED (wstatus))
|
||
exit (WEXITSTATUS (wstatus));
|
||
else /* if WIFSIGNALED (wstatus) */
|
||
{
|
||
raise (WTERMSIG (wstatus));
|
||
|
||
/* Just in case the signal raised doesn't cause an
|
||
exit. */
|
||
exit (127);
|
||
}
|
||
}
|
||
|
||
/* Otherwise, continue looping. */
|
||
}
|
||
}
|
||
}
|